JavaScript Closure
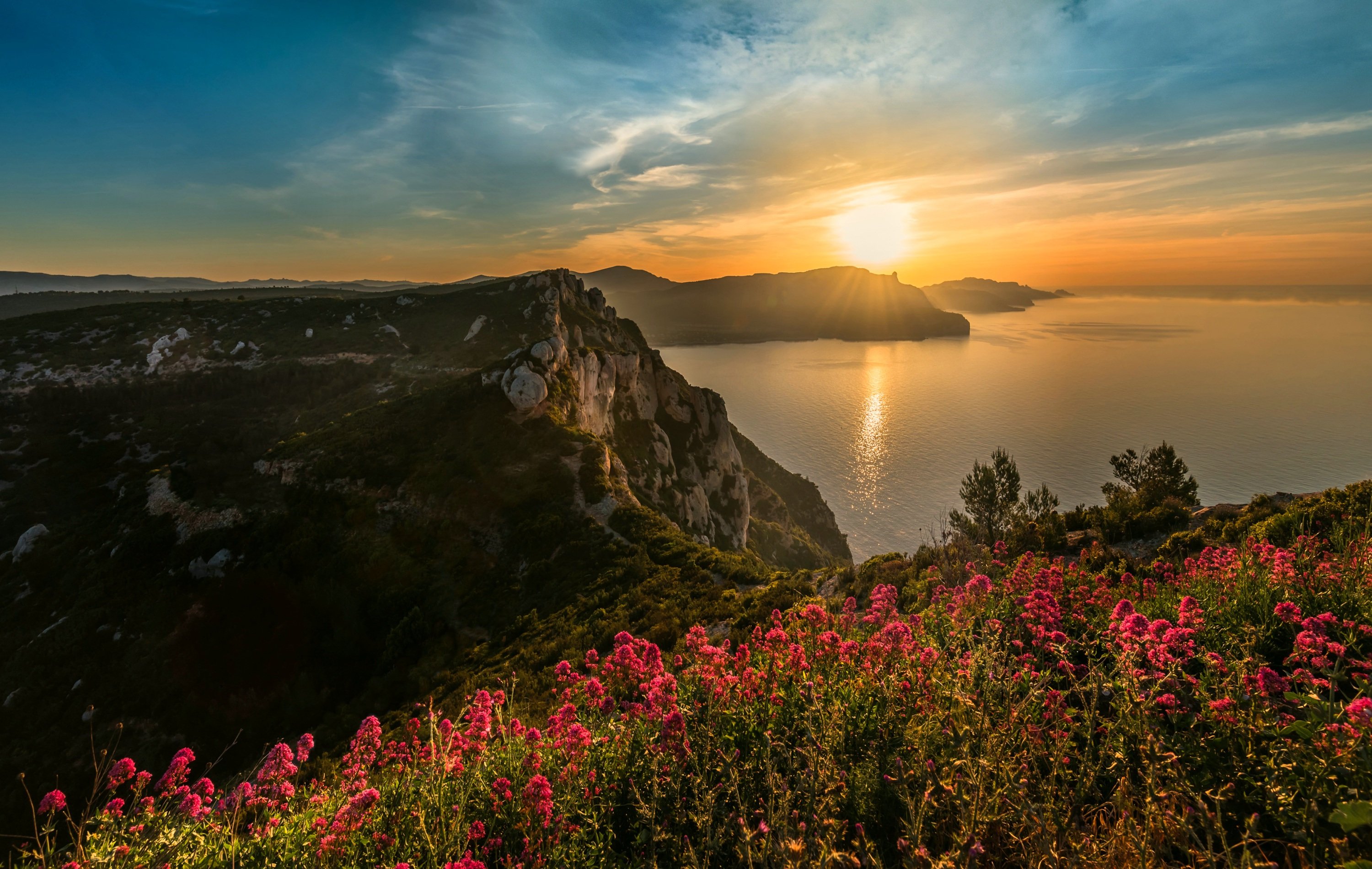
JavaScript closures are an essential concept that every developer should understand. In simple terms, closures allow developers to access and manipulate variables outside of their current scope. They are powerful tools for creating modular and efficient code, but can also be a source of confusion and unexpected behavior.
What is a closure in JavaScript ?
A closure is a function that has access to its parent function's variables, even after the parent function has returned. This is because the child function maintains a reference to the parent function's variables in memory. The concept of closure can be a bit abstract, but it becomes clearer with a simple example:
In this example, outerFunction creates a variable called outerVariable and then defines an inner function called innerFunction. innerFunction has access to outerVariable, even though it is defined outside of its own scope. When we call outerFunction, it returns innerFunction, which we assign to the variable ``closure``. When we call ``closure``, it logs the value of ``outerVariable`` to the console.
Why use closures?
Closures can be incredibly useful in JavaScript programming. One common use case is to create private variables and functions. By using a closure, we can define a variable or function within a function's scope that is not accessible from outside the function. This can help us create more secure and modular code:
In this example, ``counter`` creates a variable called ``count`` and two inner functions called ``increment`` and ``decrement``. ``increment`` and ``decrement`` have access to ``count``, even though it is not defined within their own scope. counter returns an object that exposes the ``increment`` and ``decrement`` functions. This allows us to create multiple independent counters, each with their own private ``count`` variable.
Closures can also help us create more efficient code by reducing the number of global variables. Global variables can lead to naming conflicts and make it difficult to reason about the code's behavior. By using closures to create private variables and functions, we can reduce our reliance on global variables and make our code more modular.
Potential pitfalls of closures
While closures can be incredibly useful, they can also be a source of confusion and unexpected behavior. One potential pitfall of closures is the issue of variable scope. Closures can cause variables to persist in memory even when they are no longer needed, leading to memory leaks and decreased performance.
Another potential issue with closures is that they can create unexpected interactions between variables in different scopes. This can lead to hard-to-debug errors and make it difficult to reason about the code's behavior.
Conclusion:
JavaScript closures are a powerful tool for creating more efficient and modular code. They allow developers to access and manipulate variables outside of their current scope, which can help create more secure and efficient code. However, closures can also be a source of confusion and unexpected behavior, so it is important to use them with care and consideration. By understanding closures and their potential pitfalls, developers can write better, more maintainable code.